C programming is a general-purpose, high-level programming language that was developed in the early 1970s by Dennis Ritchie. It is widely used for system programming, embedded systems, and developing software applications. C programming is known for its efficiency, portability, and flexibility, making it a popular choice for software developers.
Table of Contents
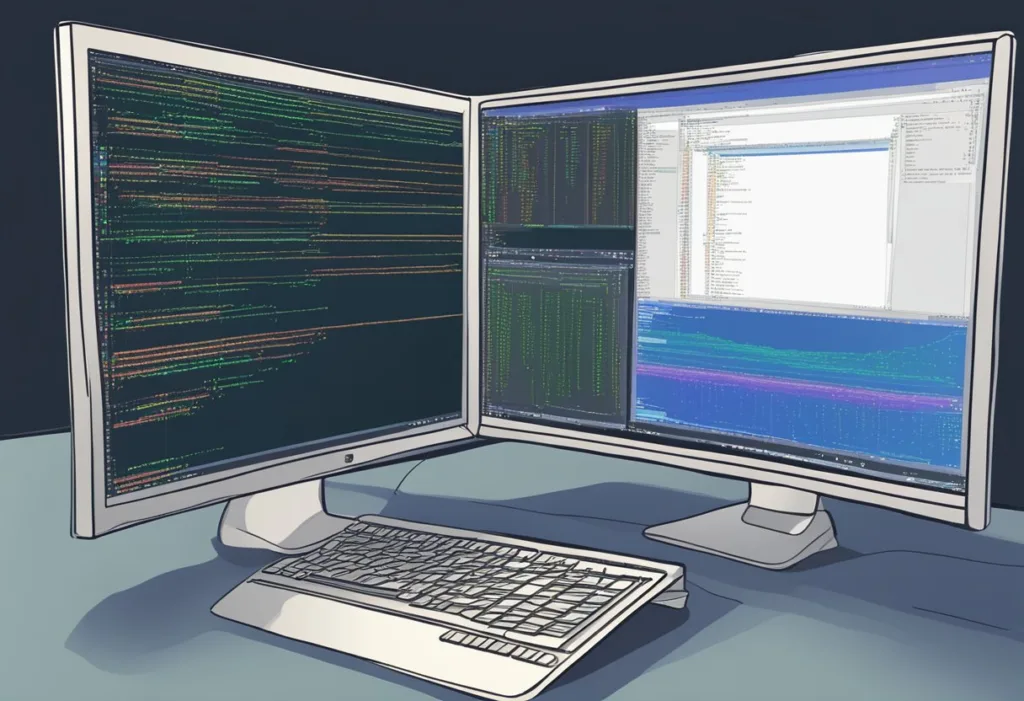
C programming provides a wide range of functionalities and features that make it a powerful tool for software development. It supports a variety of data types, including integers, floating-point numbers, characters, and arrays. C programming also includes control structures, such as loops and conditional statements, which allow developers to create complex programs with ease.
Key Takeaways
- C programming is a general-purpose, high-level programming language that is widely used for system programming, embedded systems, and developing software applications.
- C programming is known for its efficiency, portability, and flexibility, making it a popular choice for software developers.
- C programming provides a wide range of functionalities and features that make it a powerful tool for software development.
C Programming Basics
Syntax and Structure
C programming language is a compiled language that follows a specific syntax and structure. The syntax of the C language is very straightforward and easy to understand. The basic structure of a C program consists of a main function that contains the program’s instructions. Programs written in C are stored in plain text files ending in.c.
Data Types
C language has several built-in data types such as integers, characters, floating-point numbers, and arrays. Each data type has a specific size and range of values that it can store. C also allows the creation of user-defined data types using structures and unions.
Variables
Variables are used to store data in a C program. Each variable has a specific data type and a unique name. Variables can be declared and initialized at the same time or separately. In C, variables are declared at the beginning of a function or block.
Operators
C language has several operators such as arithmetic, relational, logical, bitwise, and assignment operators. Operators are used to perform operations on variables and values. For example, the addition operator (+) is used to add two values.
Control Flow
Control flow statements are used to control the flow of a C program. C language has several control flow statements such as if-else, switch-case, for loop, while loop, and do-while loop. These statements are used to make decisions and repeat a set of instructions.
Overall, understanding the basics of C programming is essential for writing efficient and effective programs. By mastering the syntax and structure, data types, variables, operators, and control flow statements, programmers can create powerful applications that can perform complex tasks.
Advanced Topics
Pointers
Pointers are one of the most powerful features of C programming. They are variables that store the memory address of another variable. Pointers allow a programmer to directly manipulate memory, which can be useful in certain situations, such as when working with large data structures. Pointers can also be used to pass variables by reference, which can save memory and improve performance.
To declare a pointer, use the *
symbol before the variable name. For example, int *ptr;
declares a pointer to an integer variable. To assign a value to a pointer, use the &
operator before the variable name. For example, int x = 5; int *ptr = &x;
assigns the memory address of x
to the pointer variable ptr
.
Memory Management
C provides low-level memory management features that allow a programmer to allocate and deallocate memory dynamically. This can be useful when working with data structures that have a variable size or when working with large amounts of data.
The malloc()
function is used to allocate memory dynamically. It takes the number of bytes to allocate as an argument and returns a pointer to the allocated memory. The free()
function is used to deallocate memory that was previously allocated with malloc()
. It takes a pointer to the memory to deallocate as an argument.
Functions
Functions are a key feature of C programming. They allow a programmer to break a large program into smaller, more manageable pieces. Functions can be used to perform a specific task or to return a value to the calling program.
To declare a function, use the return_type function_name(parameters)
syntax. For example, int add(int x, int y)
declares a function called add
that takes two integer parameters and returns an integer value. To call a function, use the function name followed by the arguments in parentheses. For example, int result = add(3, 5);
calls the add
function with arguments 3
and 5
and assigns the return value to the variable result
.
Structures and Unions
Structures and unions are data types that allow a programmer to group related data together. Structures are used to group data of different types, while unions are used to group data of the same size.
To declare a structure, use the struct
keyword followed by the structure name and a list of member variables. For example, struct person { char name[50]; int age; };
declares a structure called person
that contains a character array called name
and an integer variable called age
. To access the member variables of a structure, use the .
operator. For example, struct person p; p.age = 25;
assigns the value 25
to the age
member variable of the p
structure.
File I/O
C provides functions for reading and writing data to and from files. This can be useful when working with large amounts of data or when data needs to be stored between program runs.
To open a file, use the fopen()
function. It takes the file name and mode as arguments and returns a file pointer. For example, FILE *fp = fopen("file.txt", "w");
opens a file called file.txt
for writing and returns a file pointer called fp
. To write data to a file, use the fprintf()
function. For example, fprintf(fp, "Hello, world!");
writes the string "Hello, world!"
to the file pointed to by fp
. To close a file, use the fclose()
function. For example, fclose(fp);
closes the file pointed to by fp
.
Frequently Asked Questions
What is the primary use of C programming in modern applications?
C programming is widely used in modern applications, particularly in system programming, embedded systems, and operating systems. It is also used in developing various applications for desktop and mobile platforms. C is known for its high performance and low-level memory manipulation capabilities, making it ideal for developing software for systems with limited resources.
How can one begin learning C programming effectively?
One can begin learning C programming effectively by first understanding the basic concepts and syntax of the language. It is recommended to start with simple programs and gradually move on to more complex ones. Reading books and online tutorials can also help in learning the language effectively. It is also important to practice coding regularly to improve programming skills.
What are the basic concepts one must understand to master C programming?
To master C programming, one must have a good understanding of basic programming concepts such as variables, data types, control structures, functions, arrays, pointers, and structures. It is also important to have a good understanding of memory allocation and manipulation, as well as file handling and input/output operations.
Could you explain what a program in C consists of?
A program in C consists of various components, including preprocessor directives, functions, variables, and statements. The preprocessor directives are used to include header files and define macros. Functions are used to perform specific tasks, and variables are used to store data. Statements are used to control the flow of the program.
At which level of programming languages is C categorized?
C is categorized as a middle-level programming language, as it combines both high-level and low-level language features. It provides low-level memory manipulation capabilities, as well as high-level functions and data structures. C is known for its efficiency and speed, making it ideal for system programming.
Where can I find comprehensive resources for learning C programming?
There are many resources available online for learning C programming, including tutorials, books, and online courses. Some popular resources include GeeksforGeeks, Udemy, and Codeacademy. It is important to choose a resource that suits your learning style and pace. It is also recommended to practice coding regularly to improve programming skills.